Kotlin Type Aliases: Enhancing Code Clarity - Wednesday's Kotlin Kuppa #1
Exploring Kotlin's Type Aliases: A Dive into Clarity, Readability, and Understanding Their Boundaries
Hello, it's Mirco! Welcome to the inaugural Wednesday's Kotlin Kuppa ☕️
Every Wednesday, I'll brew a fresh, hands-on Kotlin tip just for you. Curious about my previous insights? Check out my take on Kotlin and Variance 🔗.
Don't miss out on these weekly sips—subscribe if you haven't!
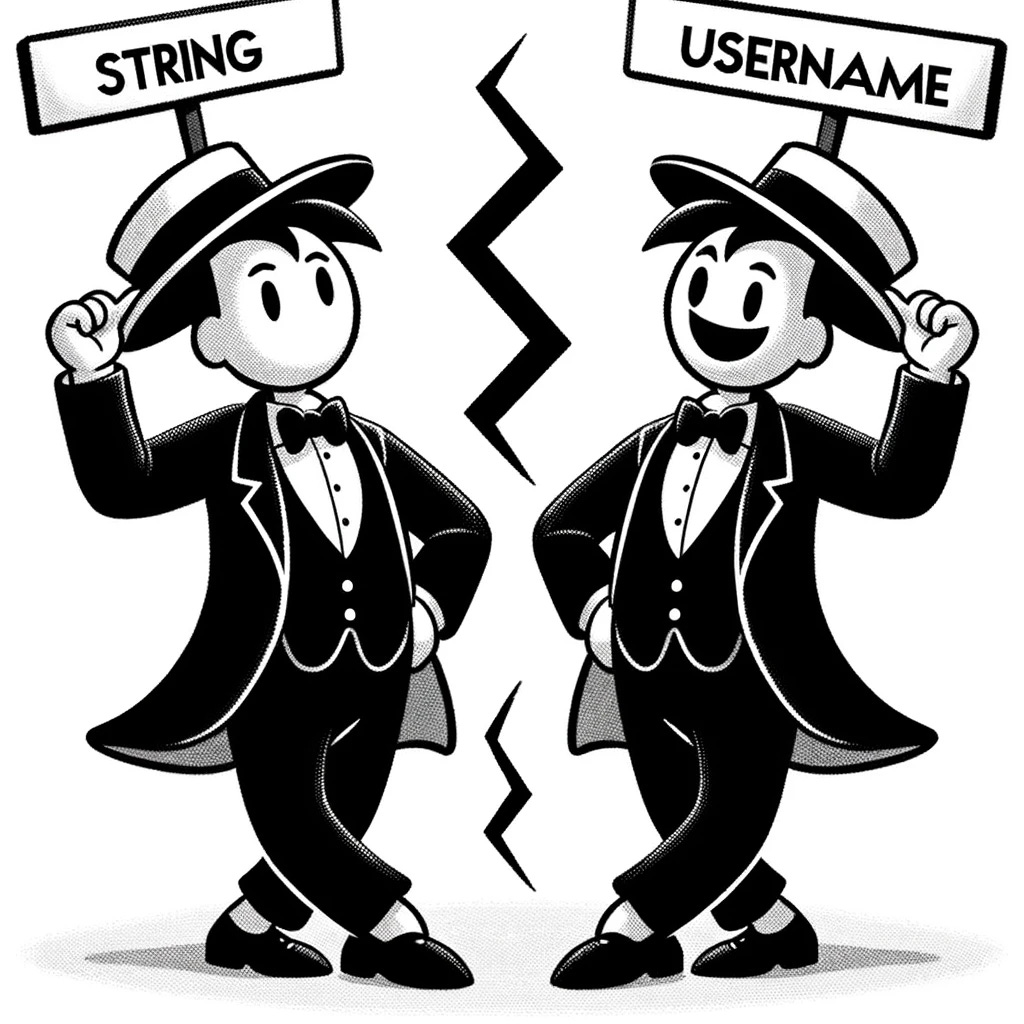
Kotlin's Type Aliases provide a simple yet effective way to enhance code readability. By allowing developers to use alternative names for existing types, they streamline complex declarations. While Type Aliases shine brightly in Kotlin, they aren't its invention. Languages like Scala, TypeScript, C++, and even Ada have embraced similar concepts. But it's interesting to note that Java, a giant in the programming world, lacks this feature 🤔.
Let's dive deeper into this intriguing and handy feature.
What is a Type Alias?
At its core, a type alias lets you give an existing type a new name, similar to how you'd use aliases in your command line to shorten lengthy commands. The magic happens with the typealias
keyword.
typealias Role = String
From this point on, Role
and String
can be used interchangeably. Role
now essentially acts as another name for String
wherever the type alias is imported.
Benefits of Using Type Aliases
Our brief overview might make type aliases seem trivial, but their impact is profound. Just take a peek at languages without this feature, like Java. You might find Java methods resembling:
public void processUserDetails(boolean isActive, boolean isVerified, boolean hasPremiumAccess, String username, int age) {
// ... method implementation
}
It's easy to jumble up the order of those boolean parameters, right? Now, imagine this with type aliases:
public void processUserDetails(IsActive isActive, IsVerified isVerified, HasPremiumAccess hasPremiumAccess, String username, int age) {
// ... method implementation
}
Kotlin's type aliases offer a level of clarity that can be challenging to achieve in languages like Java without resorting to additional constructs, such as wrapping primitives in classes. While Kotlin has other features like named parameters to enhance readability, type aliases stand out for their direct approach to simplifying complex type references.
Handling complex generic types becomes more manageable with type aliases. Imagine you're building a user management system where each user has a set of roles, and each role has a collection of permissions. You might encounter a type like this:
Map<User, Map<Role, List<Permission>>>
Avoid introducing such types if possible; a data class often suits better. But if you're working with a third-party API, you're somewhat tied. This is where type aliases come to the rescue:
typealias UserRolePermissions = Map<Role, List<Permission>>
typealias UserMapping = Map<User, UserRolePermissions>
fun getUserPermissions(): UserMapping {
// ... implementation
}
But that's not all! Type aliases have a flair for simplifying function types too. Picture an e-commerce app 🛍️ where product filtering is essential. Instead of repeating the lengthy signature (Product, FilterCriteria) -> Boolean
, embrace the type alias:
typealias ProductFilter = (Product, FilterCriteria) -> Boolean
Now, every time you set filters or pass them around, just use ProductFilter
and keep things tidy.
After exploring these examples, we can see the charm of type aliases in Kotlin. Here are their key benefits: 👇
Enhanced Code Readability: Type aliases make complex declarations more straightforward and intuitive. Especially useful when dealing with third-party APIs or when refactoring is not an option.
Versatility: Not limited to just data types, they can also simplify function signatures.
Increased Expressiveness: They allow for more descriptive and meaningful naming in your code.
Understanding the Limitations of Type Aliases
Type aliases in Kotlin are a valuable asset, enhancing the expressiveness and clarity of our code. They contribute significantly to both readability and code organization, showcasing simplicity at its best.
However, it's pivotal to understand their limitations. While they grant a new name to an existing type, they don't forge a new type. For instance, typealias Email = String
aids in readability but doesn't bolster type safety. To the compiler, it remains a string, open to any string value.
To summarize the limitations of type aliases 📌:
Don't Create New Types: They provide alternative names, not distinct types.
No Enhanced Type Safety: For example, any string can fit into
typealias Email = String
.Same Compiler Perception: The compiler interprets them as their original types.
For those situations where we seek tighter type constraints or heightened safety, Kotlin doesn't disappoint. The language offers a spectrum of features that can be used instead of type aliases 🛠️.
Delving into sealed classes or employing custom validation functions are excellent strategies. Kotlin’s value classes present a path to augmented type safety. They do encapsulate the "original" type, potentially adding a slight overhead.
Last Words
Having delved into Kotlin's Type Aliases, we've touched upon their potential to streamline our code. As always, there's much to learn and explore, and I hope this piece has shed some light on a feature that can be both helpful and intriguing.
Thank you for investing your time in reading this post! 🙏 If you found it valuable, please leave a comment 💬 and share it with your network 📢.